表单
编辑教程表单
描述
在本章中,我们将学习如何创建表单。 我们将在我们的示例中使用以下类和指令。
来自Bootstrap的form-group,form-control和btn类。
用于数据绑定的[(ngModel)]和用于跟踪我们的控制状态的NgControl指令。
NgControl是NgForm指令族中的一个,用于验证和跟踪表单元素。
ngSubmit伪指令用于处理表单的提交。
例子
下面的例子描述了如何在Angular 2中创建一个表单:
<html>
<head>
<title>Contact Form</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css">
<script src="https://atts.123.com/attachments/tuploads/angular2/es6-shim.min.js"></script>
<script src="https://atts.123.com/attachments/tuploads/angular2/system-polyfills.js"></script>
<script src="https://atts.123.com/attachments/tuploads/angular2/angular2-polyfills.js"></script>
<script src="https://atts.123.com/attachments/tuploads/angular2/system.js"></script>
<script src="https://atts.123.com/attachments/tuploads/angular2/typescript.js"></script>
<script src="https://atts.123.com/attachments/tuploads/angular2/Rx.js"></script>
<script src="https://atts.123.com/attachments/tuploads/angular2/angular2.dev.js"></script>
<script>
System.config({
transpiler: 'typescript',
typescriptOptions: { emitDecoratorMetadata: true },
packages: {'app': {defaultExtension: 'ts'}}
});
System.import('/angular2/src/app/main')
.then(null, console.error.bind(console));
</script>
</head>
<body>
<my-app>Loading...</my-app>
</body>
</html>
上述代码包括以下配置选项:
您可以使用typescript版本配置index.html文件。在使用transpiler选项运行应用程序之前,SystemJS将TypeScript转换为JavaScript。
如果在运行应用程序之前没有翻译到JavaScript,您可能会看到浏览器中隐藏的编译器警告和错误。
当设置emitDecoratorMetadata选项时,TypeScript会为代码的每个类生成元数据。如果不指定此选项,将生成大量未使用的元数据,这会影响文件大小和对应用程序运行时的影响。
Angular 2包括来自app文件夹的包,其中文件将具有.ts扩展名。
接下来它将从应用程序文件夹加载主组件文件。如果没有找到主要组件文件,那么它将在控制台中显示错误。
当Angular调用main.ts中的引导函数时,它读取Component元数据,找到“app”选择器,找到一个名为app的元素标签,并在这些标签之间加载应用程序。
要运行代码,您需要以下TypeScript(.ts)文件,您需要保存在应用程序文件夹下。
form_main.ts
import {bootstrap} from 'angular2/platform/browser';
import {AppComponent} from "./data_binding_app.component";
bootstrap(AppComponent);
contact.ts
export class Contact {
constructor(
public firstname: string,
public lastname: string,
public country: string,
public phone: number
) { }
}
Contact类包含在我们的表单中使用的名字,姓氏,国家和电话。
forms_app.component.ts
import {Component} from 'angular2/core';
import {ContactComponent} from './contact-form.component'
@Component({
selector: 'my-app',
template: '',
directives: [ContactComponent]
})
export class AppComponent { }
@Component是一个装饰器,它使用配置对象来创建组件。
选择器创建组件的实例,在父HTML中找到<my-app>标记。
该模板告诉Angular渲染为视图。
上面的app.component.ts将导入ContactComponent组件并使用指令来包含组件。
contact-form.component.ts
import {Component} from 'angular2/core';
import {NgForm} from 'angular2/common';
import { Contact } from './contact';
@Component({
selector: 'contact-form',
templateUrl: 'app/contact-form.component.html'
})
export class ContactComponent {
countries = ['India', 'Australia', 'England', 'South Africa', 'USA', 'Japan', 'Singapore'];
contact = new Contact('Ravi', 'Shankar', this.countries[0], 6445874544);
submitted = false;
onSubmit() { this.submitted = true; }
active = true;
newContact() {
this.contact = new Contact('', '');
this.active = false;
setTimeout(()=> this.active=true, 0);
}
}
导入NgForm,它提供CSS类和模型状态。
templateUrl属性提供了contact-form.component.html文件的路径,其中包含我们的表单元素。
onSubmit()方法将在调用后将提交的值更改为true,newContact()将创建新的联系人。
contact-form.component.html
<div class="container">
<div [hidden]="submitted">
<h2>Contact Form</h2>
<form *ngIf="active" (ngSubmit)="onSubmit()" #contactForm="ngForm" novalidate>
<div class="form-group">
<label for="firstname">First Name</label>
<input type="text" class="form-control" placeholder="Enter Your First Name" required
[(ngModel)]="contact.firstname"
ngControl="firstname" #firstname="ngForm" >
<div [hidden]="firstname.valid || firstname.pristine" class="alert alert-danger">
firstname is required
</div>
</div>
<div class="form-group">
<label for="lastname">Last Name</label>
<input type="text" class="form-control" placeholder="Enter Your Last Name"
[(ngModel)]="contact.lastname"
ngControl="lastname" >
</div>
<div class="form-group">
<label for="country">Country</label>
<select class="form-control" required
[(ngModel)]="contact.country"
ngControl="country" #country="ngForm" >
<option value="" selected disabled>Select Your Country</option>
<option *ngFor="#coun of countries" [value]="coun">{{coun}}</option>
</select>
<div [hidden]="country.valid || country.pristine" class="alert alert-danger">
Country is required
</div>
</div>
<div class="form-group">
<label for="phone">Phone Number</label>
<input type="number" class="form-control" placeholder="Enter Your Phone Number"
[(ngModel)]="contact.phone"
ngControl="phone"
>
</div>
<button type="submit" class="btn btn-primary" [disabled]="!contactForm.form.valid">Submit</button>
<button type="button" class="btn btn-primary" (click)="newContact()">New Contact</button>
</form>
</div>
<div [hidden]="!submitted">
<h2>Your contact details :</h2>
<div class="well">
<div class="row">
<div class="col-xs-2">First Name</div>
<div class="col-xs-10 pull-left">{{ contact.firstname }}</div>
</div>
<div class="row">
<div class="col-xs-2">Last Name</div>
<div class="col-xs-10 pull-left">{{ contact.lastname }}</div>
</div>
<div class="row">
<div class="col-xs-2">Country</div>
<div class="col-xs-10 pull-left">{{ contact.country }}</div>
</div>
<div class="row">
<div class="col-xs-2">Phone Number</div>
<div class="col-xs-10 pull-left">{{ contact.phone }}</div>
</div>
<br>
<button class="btn btn-primary" (click)="submitted=false">Edit Contact</button>
</div>
</div>
</div>
上面的代码包含表单,在提交表单时,ngSubmit指令调用onSubmit()方法。
当提交的设置为false时,显示联系人详细信息。
它使用原始和有效的验证表单输入元素。
ngIf指令检查活动对象是否设置为true以显示表单。
输出
让我们执行以下步骤,看看上面的代码如何工作:
将上面的HTML代码保存为index.html文件,如同我们在环境章节中创建的,并使用上面的包含.ts文件的应用程序文件夹。
打开终端窗口并输入以下命令:
npm start
稍后,浏览器选项卡应打开并显示输出

选择支付方式:
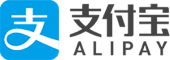
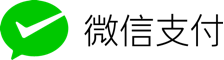
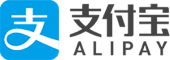
备注:
转账时请填写正确的金额和备注信息,到账由人工处理,可能需要较长时间