Java – 将 Array 转成 Stream
编辑教程在Java 8里,你可以用Arrays.stream
或 Stream.of
来将数组转换成流。
1. 对象数组
对于对象数组,Arrays.stream
和 Stream.of
方法都可以返回同样的输出结果。
TestJava8.java
package com.mkyong.java8;
import java.util.Arrays;
import java.util.stream.Stream;
public class TestJava8 {
public static void main(String[] args) {
String[] array = {"a", "b", "c", "d", "e"};
//Arrays.stream
Stream<string> stream1 = Arrays.stream(array);
stream1.forEach(x -> System.out.println(x));
//Stream.of
Stream<string> stream2 = Stream.of(array);
stream2.forEach(x -> System.out.println(x));
}
}
Output
a
b
c
d
e
a
b
c
d
e
让我们看一下JDK的源码。
Arrays.java
/**
* Returns a sequential {@link Stream} with the specified array as its
* source.
*
* @param <t> The type of the array elements
* @param array The array, assumed to be unmodified during use
* @return a {@code Stream} for the array
* @since 1.8
*/
public static <t> Stream<t> stream(T[] array) {
return stream(array, 0, array.length);
}
Stream.java
/**
* Returns a sequential ordered stream whose elements are the specified values.
*
* @param <t> the type of stream elements
* @param values the elements of the new stream
* @return the new stream
*/
@SafeVarargs
@SuppressWarnings("varargs") // Creating a stream from an array is safe
public static<t> Stream<t> of(T... values) {
return Arrays.stream(values);
}
请注意
对于对象数组,Stream.of
方法会在内部调用Arrays.stream
。
2. 原始数组
对于原始数组,Arrays.stream
和 Stream.of
会返回不同的输出结果。
TestJava8.java
package com.mkyong.java8;
import java.util.Arrays;
import java.util.stream.IntStream;
import java.util.stream.Stream;
public class TestJava8 {
public static void main(String[] args) {
int[] intArray = {1, 2, 3, 4, 5};
// 1. Arrays.stream -> IntStream
IntStream intStream1 = Arrays.stream(intArray);
intStream1.forEach(x -> System.out.println(x));
// 2. Stream.of -> Stream<int[]>
Stream<int[]> temp = Stream.of(intArray);
// Cant print Stream<int[]> directly, convert / flat it to IntStream
IntStream intStream2 = temp.flatMapToInt(x -> Arrays.stream(x));
intStream2.forEach(x -> System.out.println(x));
}
}
Output
1
2
3
4
5
1
2
3
4
5
让我们看一下JDK的源码。
Arrays.java
/**
* Returns a sequential {@link IntStream} with the specified array as its
* source.
*
* @param array the array, assumed to be unmodified during use
* @return an {@code IntStream} for the array
* @since 1.8
*/
public static IntStream stream(int[] array) {
return stream(array, 0, array.length);
}
Stream.java
/**
* Returns a sequential {@code Stream} containing a single element.
*
* @param t the single element
* @param <t> the type of stream elements
* @return a singleton sequential stream
*/
public static<t> Stream<t> of(T t) {
return StreamSupport.stream(new Streams.StreamBuilderImpl<>(t), false);
}
该用哪一个呢?
对于对象数组,都会调用Arrays.stream
(参见示例1,JDK源代码)。
对于原始数组,更推荐Arrays.stream
,因为它直接返回固定大小的IntStream
,更易于操作。
P.S 使用Oracle JDK 1.8.0_77版本测试
References
Mos固件,小电视必刷固件
ES6 教程
Vue.js 教程
JSON 教程
jQuery 教程
HTML 教程
HTML 5 教程
CSS 教程
CSS3 教程
JavaScript 教程
DHTML 教程
JSON在线格式化工具
JS在线运行
JSON解析格式化
jsfiddle中国国内版本
JS代码在线运行
PHP代码在线运行
Java代码在线运行
C语言代码在线运行
C++代码在线运行
Python代码在线运行
Go语言代码在线运行
C#代码在线运行
JSRUN闪电教程系统是国内最先开创的教程维护系统, 所有工程师都可以参与共同维护的闪电教程,让知识的积累变得统一完整、自成体系。
大家可以一起参与进共编,让零散的知识点帮助更多的人。
X

选择支付方式:
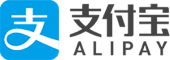
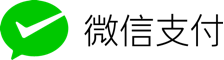
立即支付
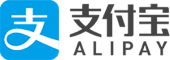
¥
9.99
无法付款,请点击这里
金额: 0 元
备注:
转账时请填写正确的金额和备注信息,到账由人工处理,可能需要较长时间
备注:
转账时请填写正确的金额和备注信息,到账由人工处理,可能需要较长时间
如有疑问请联系QQ:565830900
正在生成二维码, 此过程可能需要15秒钟