Java – How to add days to current date
编辑教程This article shows you how to add days to the current date, using the classic java.util.Calendar
and the new Java 8 date and time APIs.
1. Calendar.add
Example to add 1 year, 1 month, 1 day, 1 hour, 1 minute and 1 second to the current date.
DateExample.java
package com.mkyong.time;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class DateExample {
private static final DateFormat dateFormat = new SimpleDateFormat("yyyy/MM/dd HH:mm:ss");
public static void main(String[] args) {
Date currentDate = new Date();
System.out.println(dateFormat.format(currentDate));
// convert date to calendar
Calendar c = Calendar.getInstance();
c.setTime(currentDate);
// manipulate date
c.add(Calendar.YEAR, 1);
c.add(Calendar.MONTH, 1);
c.add(Calendar.DATE, 1); //same with c.add(Calendar.DAY_OF_MONTH, 1);
c.add(Calendar.HOUR, 1);
c.add(Calendar.MINUTE, 1);
c.add(Calendar.SECOND, 1);
// convert calendar to date
Date currentDatePlusOne = c.getTime();
System.out.println(dateFormat.format(currentDatePlusOne));
}
}
Output
2016/11/10 17:11:48
2017/12/11 18:12:49
2. Java 8 Plus Minus
In Java 8, you can use the plus and minus methods to manipulate LocalDate, LocalDateTime and ZoneDateTime, see the following examples
LocalDateTimeExample.java
package com.mkyong.time;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
import java.util.Date;
public class LocalDateTimeExample {
private static final String DATE_FORMAT = "yyyy/MM/dd HH:mm:ss";
private static final DateFormat dateFormat = new SimpleDateFormat(DATE_FORMAT);
private static final DateTimeFormatter dateFormat8 = DateTimeFormatter.ofPattern(DATE_FORMAT);
public static void main(String[] args) {
// Get current date
Date currentDate = new Date();
System.out.println("date : " + dateFormat.format(currentDate));
// convert date to localdatetime
LocalDateTime localDateTime = currentDate.toInstant().atZone(ZoneId.systemDefault()).toLocalDateTime();
System.out.println("localDateTime : " + dateFormat8.format(localDateTime));
// plus one
localDateTime = localDateTime.plusYears(1).plusMonths(1).plusDays(1);
localDateTime = localDateTime.plusHours(1).plusMinutes(2).minusMinutes(1).plusSeconds(1);
// convert LocalDateTime to date
Date currentDatePlusOneDay = Date.from(localDateTime.atZone(ZoneId.systemDefault()).toInstant());
System.out.println("\nOutput : " + dateFormat.format(currentDatePlusOneDay));
}
}
Output
date : 2016/11/10 17:40:11
localDateTime : 2016/11/10 17:40:11
Output : 2017/12/11 18:41:12
References
Mos固件,小电视必刷固件
ES6 教程
Vue.js 教程
JSON 教程
jQuery 教程
HTML 教程
HTML 5 教程
CSS 教程
CSS3 教程
JavaScript 教程
DHTML 教程
JSON在线格式化工具
JS在线运行
JSON解析格式化
jsfiddle中国国内版本
JS代码在线运行
PHP代码在线运行
Java代码在线运行
C语言代码在线运行
C++代码在线运行
Python代码在线运行
Go语言代码在线运行
C#代码在线运行
JSRUN闪电教程系统是国内最先开创的教程维护系统, 所有工程师都可以参与共同维护的闪电教程,让知识的积累变得统一完整、自成体系。
大家可以一起参与进共编,让零散的知识点帮助更多的人。
X

选择支付方式:
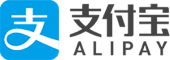
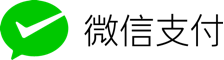
立即支付
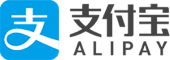
¥
9.99
无法付款,请点击这里
金额: 0 元
备注:
转账时请填写正确的金额和备注信息,到账由人工处理,可能需要较长时间
备注:
转账时请填写正确的金额和备注信息,到账由人工处理,可能需要较长时间
如有疑问请联系QQ:565830900
正在生成二维码, 此过程可能需要15秒钟