JSONP获取文章数
编辑教程JSONP获取Twitter和Facebook文章数的具体步骤
Twitter和Facebook要求身份验证来获取文章的数量,但事实证明你可以通过JSONP来获取这些信息,本文介绍如何使用一些简单的代码来获取并跳过验证这一步,请参考下面的步骤。
JavaScript代码
我将使用基本的JavaScript来告诉你如何做到这一点:
代码如下:
// 获取文章数量的封装对象
var socialGetter = (function() {
/* JSONP: 获取脚本的工具函数 */
function injectScript(url) {
var script = document.createElement('script');
script.async = true;
script.src = url;
document.body.appendChild(script);
}
return {
getFacebookCount: function(url, callbackName) {
injectScript('https://graph.facebook.com/?id=' + url + '&callback=' + callbackName);
},
getTwitterCount: function(url, callbackName) {
injectScript('http://urls.api.twitter.com/1/urls/count.json?url=' + url + '&callback=' + callbackName);
}
};
})();
// 回调方法
function twitterCallback(result) {
result.count && console.log('The count is: ', result.count);
}
function facebookCallback(result) {
result.shares && console.log('The count is: ', result.shares);
}
// 调用
socialGetter.getFacebookCount('http://davidwalsh.name/twitter-facebook-jsonp', 'facebookCallback');
socialGetter.getTwitterCount('http://davidwalsh.name/twitter-facebook-jsonp', 'twitterCallback');
因为有众多轻量级的 micro-frameworks来处理JSONP,所以本文最重要的部分可能是获取信息的url了。根据需要和习惯选择你的JSONP工具!
Twitter和Facebook对于这些请求肯定有数量和频率上的限制,所以如果你的网站访问量很大,则JSONP很可能会被拦截或屏蔽。
一种快速的解决方案是将文章数量信息存储在sessionStorage中,但这只是针对单个用户的方式。如果你运行的网站流量较大,你最好选择在服务器端抓取数据并缓存下来,并在一定的时间内自动刷新。
国外社交网站获取分享数量APIs
GET URL:
http://cdn.api.twitter.com/1/urls/count.JSon?url=http://stylehatch.co
返回结果:
{
"count":528,
"url":"http://stylehatch.co/"
}
GET URL:
http://graph.facebook.com/?id=http://stylehatch.co
返回结果:
{
"id": "http://stylehatch.co",
"shares": 61
}
GET URL:
http://api.pinterest.com/v1/urls/count.json?callback=&url=http://stylehatch.co
返回结果:
({"count": 0, "url": "http://stylehatch.co"})
GET URL:
http://www.linkedin.com/countserv/count/share?url=http://stylehatch.co&format=json
返回结果:
{
"count":17,
"fCnt":"17",
"fCntPlusOne":"18",
"url":"http:\/\/stylehatch.co"
}
Google Plus
POST URL:
https://clients6.google.com/rpc?key=YOUR_API_KEY
POST body:
[{
"method":"pos.plusones.get",
"id":"p",
"params":{
"nolog":true,
"id":"http://stylehatch.co/",
"source":"widget",
"userId":"@viewer",
"groupId":"@self"
},
"jsonrpc":"2.0",
"key":"p",
"apiVersion":"v1"
}]
返回结果:
[{
"result": {
"kind": "pos#plusones",
"id": "http://stylehatch.co/",
"isSetByViewer": false,
"metadata": {
"type": "URL",
"globalCounts": {
"count": 3097.0
}
}
} ,
"id": "p"
}]
StumbledUpon
GET URL:
http://www.stumbleupon.com/services/1.01/badge.getinfo?url=http://stylehatch.co
返回结果:
{
"result":{
"url":"http:\/\/stylehatch.co\/",
"in_index":true,
"publicid":"1iOLcK",
"views":39,
"title":"Style Hatch - Hand Crafted Digital Goods",
"thumbnail":"http:\/\/cdn.stumble-upon.com\/mthumb\/941\/72725941.jpg",
"thumbnail_b":"http:\/\/cdn.stumble-upon.com\/bthumb\/941\/72725941.jpg",
"submit_link":"http:\/\/www.stumbleupon.com\/submit\/?url=http:\/\/stylehatch.co\/",
"badge_link":"http:\/\/www.stumbleupon.com\/badge\/?url=http:\/\/stylehatch.co\/",
"info_link":"http:\/\/www.stumbleupon.com\/url\/stylehatch.co\/"
},
"timestamp":1336520555,
"success":true
}
这里有一个网站封装了一个小插件,专门用来在页面上显示社交网站分享工具条,可以直接拿过来用,比较方便!http://sharrre.com/
Facebook,Twitter,LinkedIn比较常用,给出调用API的例子:
// Facebook
$.getJSON("http://graph.facebook.com/?id=http://stylehatch.co", function (d) {
$("#fackbook_count").text("The Facebook Share count is: " + d.shares);
});
// Twitter
$.getJSON("http://cdn.api.twitter.com/1/urls/count.json?url=http://stylehatch.co&callback=?", function (d) {
$("#twitter_count").text("The Twitter Share count is: " + d.count);
});
// LinkedIn
$.getJSON("http://www.linkedin.com/countserv/count/share?url=http://stylehatch.co&callback=?", function (d) {
$("#linkedin_count").text("The LinkdeIn Share count is: " + d.count);
});
IE浏览器可能会无法正确获取到Facebook返回的数据,可以尝试在URL后面加上&callback=?,这样JQuery会将它当成JSONP来调用。
Facebook还有另一个API返回XML数据,调用方法是这样的:
$.get("http://api.facebook.com/method/links.getStats?urls=http://stylehatch.co", function (d) {
$("#fackbook_count").text("The Facebook Share count is: " + $(d).find("total_count").text());
});
如果在IE浏览器下出现No Transport错误而无法获取到Facebook返回的数据,尝试在JavaScript代码的最前面加上$.support.cors = true;允许跨域访问数据。
将代码进行封装
我们将上面Facebook,Twitter,LinkedIn三个社交网站的API进行封装,以方便页面调用。
$.fn.getShareCount = function (url) {
var self = this;
var displayShareCount = function (val, obj) {
if (!isNaN(val) && val > 0) {
obj.show();
if (val > 999) {
obj.attr("title", val);
obj.text("500+");
}
else
obj.text(val);
}
};
return {
facebook: function () {
$.get("http://api.facebook.com/method/links.getStats?urls=" + url, function (d) {
var c = $(d).find("total_count").text();
self.each(function () { displayShareCount(c, $(this)); });
});
},
twitter: function () {
$.getJSON("http://cdn.api.twitter.com/1/urls/count.json?url=" + url + "&callback=?", function (d) {
self.each(function () { displayShareCount(d.count, $(this)); });
});
},
linkedin: function () {
$.getJSON("http://www.linkedin.com/countserv/count/share?url=" + url + "&callback=?", function (d) {
self.each(function () { displayShareCount(d.count, $(this)); });
});
}
};
};
然后在页面上这样调用:
$(function () {
var shareUrl = window.location.href.toLowerCase();
$('#fackbook_count').getShareCount(shareUrl).facebook();
$('#twitter_count').getShareCount(shareUrl).twitter();
$('#linkedin_count').getShareCount(shareUrl).linkedin();
});

选择支付方式:
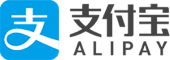
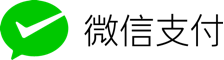
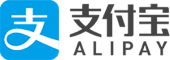
备注:
转账时请填写正确的金额和备注信息,到账由人工处理,可能需要较长时间